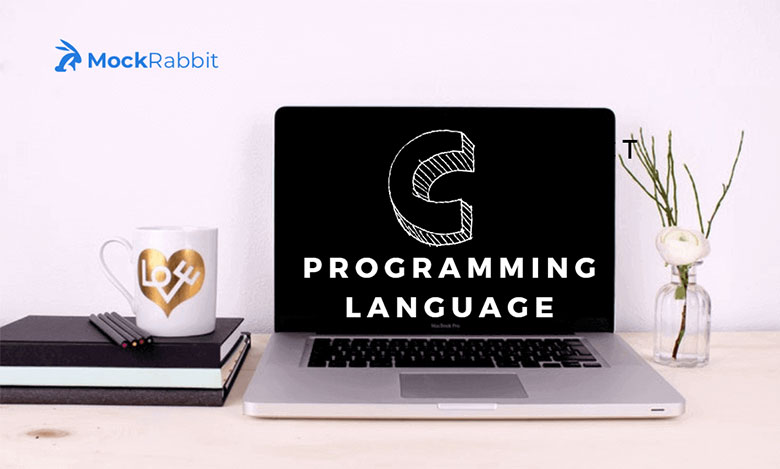
C Programming Language
The C programming language is a popular and widely used programming language for creating computer programs. The main feature of C language includes low-level access to memory, a simple set of keywords, and clean style these features make C language more suitable for system programmings like operating system and compiler development.
If you are a programmer, or if you are interested in becoming a programmer then C language is one of the powerful languages with features like reliability, portability, flexibility, interactivity, modularity, efficiency and effectiveness.
C Programming Language Interview Questions
- What are bit fields in C?
- List some applications of C programming language?
- What is the difference between a constant pointer and a constant variable?
- Compare array data type to pointer data type
- Differentiate call by value and call by reference?
- What is the best way to store flag values in a program?
- What do you understand by normalization of pointers?
- When can you use a pointer with a function?
- What is a structure in C Language.How to initialise a structure in C?
- If the size of int data type is two bytes, what is the range of signed int data type?
- When can a far pointer be used?
- Explain Enumerated types in C language?
- What is a scope resolution operator in C?
- Explain bit masking in C?
- What are Derived data types in C?
- What are static variables in C?
- What is the register variable in C language?
- Is there any data type in C with variable size?
- What is recursion in C?
- Is there any demerits of using pointer?
- Explain about block scope in C?
- What are different storage class specifiers in C
- What does paintf do?
- Explain the main function in C?
- How can you find the exact size of a data type in C?
- Explain C preprocessor?
- What is the difference between structure and union?
- Explain Pointers in C programming?
- What is the difference between #include <header file> and #include “header file” ?
- What is a NULL pointer?
- What is the use of extern in C?
- What is an auto variable in C?
- What is a void pointer?
- Explain continue keyword in C
- List some basic data types in C?
- What is a pointer on pointer?
- Distinguish between malloc() & calloc() memory allocation.
- What is keyword auto for?
- What are the valid places for the keyword break to appear?
- Explain the syntax for loop.
- What is the difference between including the header file with-in angular braces < > and double quotes “ “
- How a negative integer is stored.
- What is a static variable?
- What is a NULL pointer?
- What is the purpose of extern storage specifier?
- Explain the purpose of the function sprintf().
- What is the meaning of base address of the array?
- When should we use the register storage specifier?
- S++ or S = S+1, which can be recommended to increment the value by 1 and why?
- What is a dangling pointer?
- What is the purpose of the keyword typedef?
- What is lvalue and rvalue?
- What is the difference between actual and formal parameters?
- Can a program be compiled without main() function?
- What is the advantage of declaring void pointers?
- Where an automatic variable is stored?
- What is a nested structure?
- What is the difference between variable declaration and variable definition?
- What is a self-referential structure?
- Does a built-in header file contains built-in function definition?
- Explain modular programming.
- What is a token?
- What is a preprocessor?
- Explain the use of %i format specifier w.r.t scanf().
- How can you print a \ (backslash) using any of the printf() family of functions.
- Does a break is required by default case in switch statement?
- When to user -> (arrow) operator.
- What are bit fields?
- What are command line arguments?
- What are the different ways of passing parameters to the functions? Which to use when?
- What is the purpose of built-in stricmp() function.
- Describe the file opening mode “w+”.
- Where the address of operator (&) cannot be used?
- Is FILE a built-in data type?
- What is reminder for 5.0 % 2?
- How many operators are there under the category of ternary operators?
- Which key word is used to perform unconditional branching?
- What is a pointer to a function? Give the general syntax for the same.
- Explain the use of comma operator (,).
- What is a NULL statement?
- What is a static function?
- Which compiler switch to be used for compiling the programs using math library with gcc compiler?
- Which operator is used to continue the definition of macro in the next line?
- Which operator is used to receive the variable number of arguments for a function?
- What is the problem with the following coding snippet?
- Which built-in library function can be used to re-size the allocated dynamic memory?
- Define an array.
- What are enumerations?
- Which built-in function can be used to move the file pointer internally?
- What is a variable?
- Who designed C programming language?
- C is successor of which programming language?
- What is the full form of ANSI?
- Which operator can be used to determine the size of a data type or variable?
- Can we assign a float variable to a long integer variable?
- Is 068 a valid octal number?
- What it the return value of a relational operator if it returns any?
- How does bitwise operator XOR works.
- What is an infinite loop?
- Can variables belonging to different scope have same name? If so show an example.
- What is the default value of local and global variables?
- Can a pointer access the array?
- What are valid operations on pointers?
- What is a string length?
- What is the built-in function to append one string to another?
- Which operator can be used to access union elements if union variable is a pointer variable?
- Explain about ‘stdin’.
- Name a function which can be used to close the file stream.
- What is the purpose of #undef preprocessor?
- Define a structure.
- Name the predefined macro which be used to determine whether your compiler is ANSI standard or not?
- What is typecasting?
- What is recursion?
- Which function can be used to release the dynamic allocated memory?
- What is the first string in the argument vector w.r.t command line arguments?
- How can we determine whether a file is successfully opened or not using fopen() function?
- What is the output file generated by the linker.
- What is the maximum length of an identifier?
- What is the default function call method?
- Functions must and should be declared. Comment on this.
- When the macros gets expanded?
- Can a function return multiple values to the caller using return reserved word?
- What is a constant pointer?
- To make pointer generic for which date type it need to be declared?
- Can the structure variable be initialized as soon as it is declared?
- Is there a way to compare two structure variables?
- Which built-in library function can be used to match a patter from the string?
- What is difference between far and near pointers?
- Can we nest comments in a C code?
- Which control loop is recommended if you have to execute set of statements for fixed number of times?
- What is a constant?
- Can we use just the tag name of structures to declare the variables for the same?
- Can the main() function left empty?
- Can one function call another?